Primitive types
There are 8 primitive data types in the Java language. Understanding these data types is important.
The 8 primitive types:
- boolean
- byte
- short
- char
- int
- long
- float
- double
Okay, so we know the names of these data types but what actually are they?
To start us off we should look at the two smallest data types, the bit and the byte.
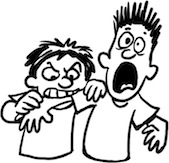
A bit is the smallest unit of data it is a single binary value that is either 0 or 1. The bit is the first building block.
Byte:
A byte is a fundamental unit of memory, it consists of 8 bits. In Java, bytes are used to save digits in memory.- Minimum value: -128
- Maximum value: 127
- Why bytes? Bytes are an excellent data type to save storage space when using small numbers in arrays. Since a byte only needs enough space to hold digits between -128 to 127
Short:
A short is a 16-bit data type. It's double the size of a byte.- Minimum value: -32,768
- Maximum value: 32,767
- Why shorts? Again, shorts are small data types, not requiring any more space than needed to hold a digit.
Int:
An int is probably the most well-known data types across programming languages. The int is a 32-bit data type. It is the default value for digits used unless there are specific memory related requirements.- Minimum value: -2,147,483,648
- Maximum value: 2,147,483,647
- Why int? The most widely recognized data type and can hold fairly large digits in memory.
Long:
Now we are dealing with the big boys. The long data type is twice the size of an int. Long is a 64-bit data type.- Minimum value: -9,223,372,036,854,775,808
- Maximum value: 9,223,372,036,854,775,807
- Why long? Used if the number range is greater than the int range.
Float:
Floats are values that have a decimal point after a digit. Floats are single-precision 32-bit IEEE 754.- Minimum value: -9,223,372,036,854,775,808
- Maximum value: 9,223,372,036,854,775,807
- Why float? To use numbers with decimal points.
Double:
Doubles are twice the size of a float. Doubles are double-precision 64-bit IEEE 754.- Minimum value: -9,223,372,036,854,775,808
- Maximum value: 9,223,372,036,854,775,807
- Why double? Doubles tend to be the default data-type when dealing with decimal digits.
Char:
Char's refer to uni-code characters. Uni-code characters include alphabets and common symbols such as (?, %, $, #, @ and more...). Char data-types are 16-bit.- Why char? For any values that are not digits.
Boolean:
I saved the smallest for last. The Boolean is 1 bit. It has only two possible values 0 (False) or 1 (True).- boolean a = false;
- boolean b = true;
- Why boolean? Booleans are important in creating logical flows in programs.
So that's all for the primitive types in Java and a good first step in understanding Java.